현재 날짜와 시간 가져오기
<!doctype html>
<html lang="ko">
<head>
<meta charset="utf-8">
<title>JavaScript</title>
<style>
body {
font-family: Consolas, monospace;
font-size: 20px;
}
</style>
</head>
<body>
<script>
var today = new Date();
document.write( '<p>' + today + '</p>' );
</script>
</body>
</html>
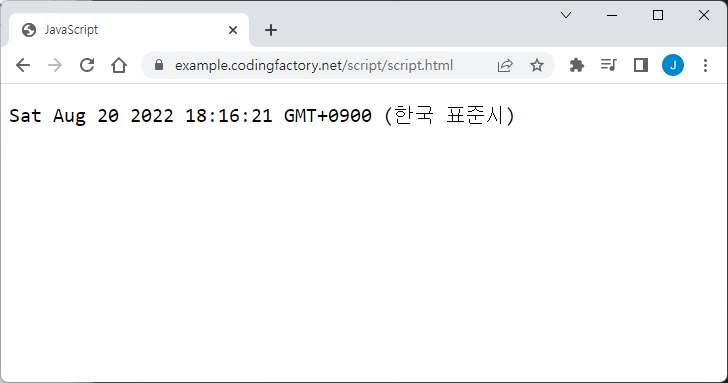
년, 월, 일, 요일 추출하기
- 년은 .getFullYear로, 월은 .getMonth로, 일은 .getDate로, 요일은 .getDay로 추출한다.
- .getMonth는 1월은 0, 2월은 1, ..., 12월은 11로 표현하므로, 1을 더해줘야 한다.
- .getDay는 일요일은 0, 월요일은 1, ..., 토요일은 6으로 표현한다.
<!doctype html>
<html lang="ko">
<head>
<meta charset="utf-8">
<title>JavaScript</title>
<style>
body {
font-family: Consolas, monospace;
font-size: 20px;
}
</style>
</head>
<body>
<script>
var today = new Date();
document.write( '<p>' + today + '</p>' );
var todayYear = today.getFullYear();
document.write( '<p>' + todayYear + '</p>' );
var todayMonth = today.getMonth() + 1;
document.write( '<p>' + todayMonth + '</p>' );
var todayDate = today.getDate();
document.write( '<p>' + todayDate + '</p>' );
var todayDay = today.getDay();
document.write( '<p>' + todayDay + '</p>' );
</script>
</body>
</html>
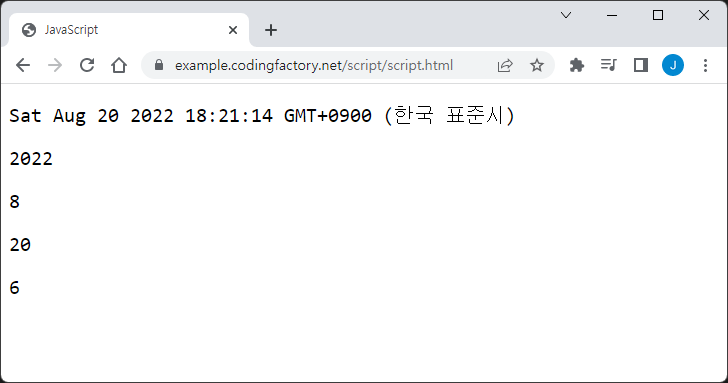
- 예를 들어 8월을 08로 나타내고 싶다면 slice를 이용한다.
<!doctype html>
<html lang="ko">
<head>
<meta charset="utf-8">
<title>JavaScript</title>
<style>
body {
font-family: Consolas, monospace;
font-size: 20px;
}
</style>
</head>
<body>
<script>
var today = new Date();
document.write( '<p>' + today + '</p>' );
var todayMonth = ( '0' + ( today.getMonth() + 1 ) ).slice( -2 );
document.write( '<p>' + todayMonth + '</p>' );
</script>
</body>
</html>
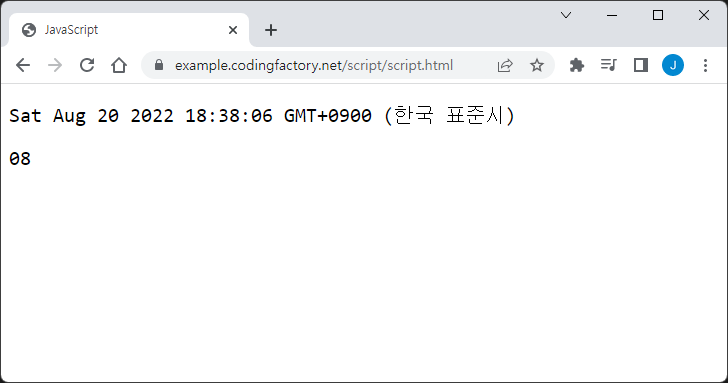
시, 분, 초 등 추출하기
- 시는 .getHours로, 분은 .getMinutes로, 초는 .getSeconds로, 밀리세컨드(천분의 일초)는 .getMilliseconds로 추출한다.
<!doctype html>
<html lang="ko">
<head>
<meta charset="utf-8">
<title>JavaScript</title>
<style>
body {
font-family: Consolas, monospace;
font-size: 20px;
}
</style>
</head>
<body>
<script>
var today = new Date();
document.write( '<p>' + today + '</p>' );
var todayHours = today.getHours();
document.write( '<p>' + todayHours + '</p>' );
var todayMinutes = today.getMinutes();
document.write( '<p>' + todayMinutes + '</p>' );
var todaySeconds = today.getSeconds();
document.write( '<p>' + todaySeconds + '</p>' );
var todayMilliseconds = today.getMilliseconds();
document.write( '<p>' + todayMilliseconds + '</p>' );
</script>
</body>
</html>
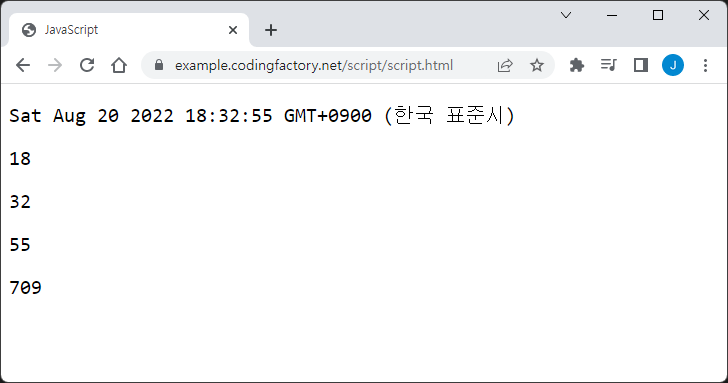